Thank You
Request for call back
What is Joi? In NodeJS
The Node.js runtime environment is extremely popular for creating server-side applications and APIs. Even though it allows developers to create various apps, security and integrity are fundamental issues. Here is where the renowned validation library JOI comes in handy. With JOI, you can logically define sanitization and validation, making the solution intuitive and user-friendly. In this article, we dig deep into JOI in nodejs validator and how it can be used to validate data.
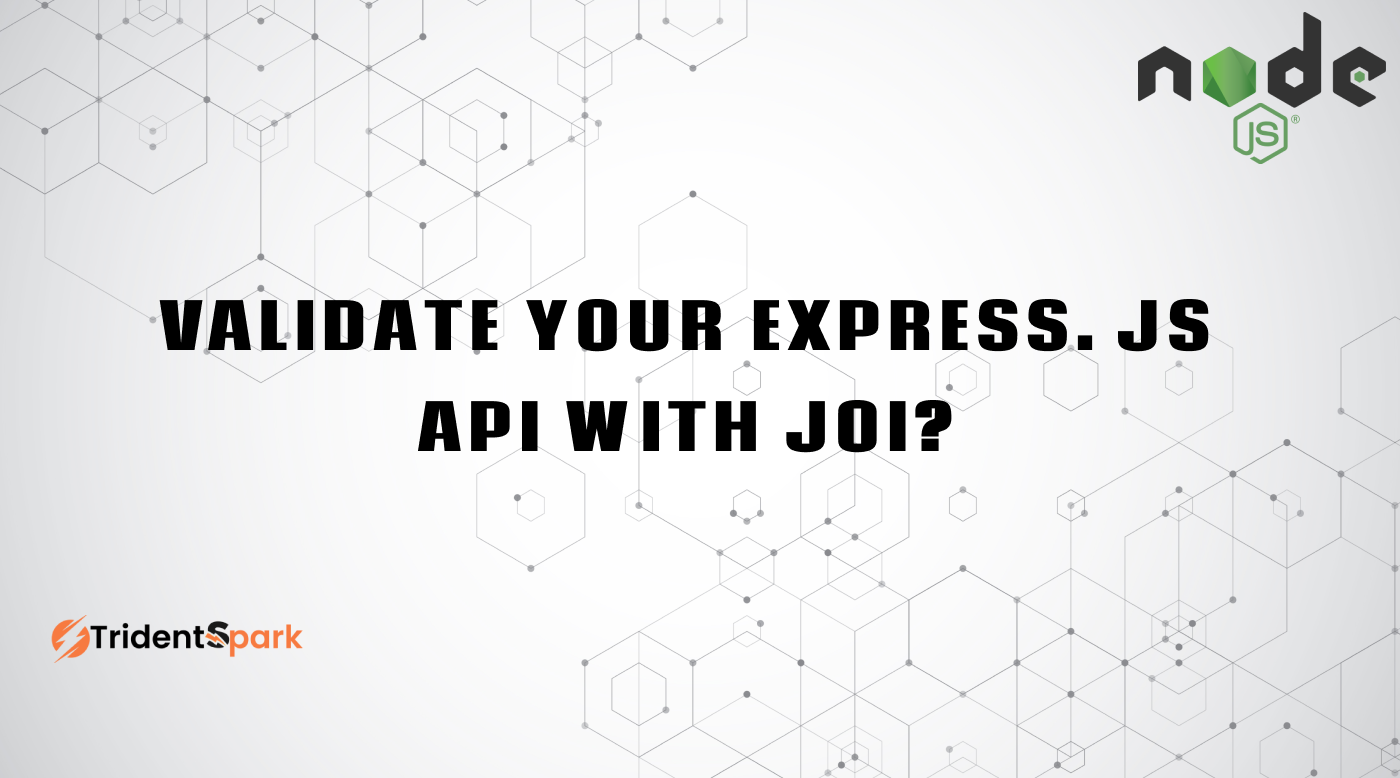
Understanding JOI
Joi is the most famous, efficient, and widely used package for object schema descriptions and validation. Joi allows the developers to build the Javascript blueprints and ensure the application accepts the accurately formatted data. With its flexible design and great features, Node.js developers will find it a valuable companion.
Best practices to set up and Validate Node.js With Joi
The Joi is a schema description and javascript ip address validation. To joi validate at the request level, you can use the Joi validation library.
Import Required Modules:
npm install joi
// Import Joi in your application const Joi = require(‘joi’)
Basic Usage
Validation Rules
// Joi provides various validation rules .string() .number() .boolean() .required() .optional() .allow() .min() .max() .length() .email() .pattern() .alphanum() etc.
Validating Request Data
In an Express route, validate incoming data using Joi:
Custom Error Messages
Override default error messages using .message():
Handling Validation Errors
Additional Resources
Creating a Joi Schema
The Joi schema defines the expected structure and rules of a specific data object.
By using Joi.object(), you can create a Joi schema that defines your data’s structure and validation requirements using various validation rules shown by Joi.
For example:
const exampleSchema = Joi.object({ name: Joi.string().min(3).required(), });
In the example above, there is a name property in a Joi schema. There is a Joi.string().min( 3).required() property for the name property. Names must be strings with at least 3 characters, and they must be strings.
Each field defined in your schema can have various validation constraints added using Joi.
The following example has more fields and validation constraints:
const userSchema = Joi.object({ email: Joi.string().email().required(), password: Joi.string().min(6).required(), age: Joi.number().min(18).optional(), employed: Joi.boolean().optional(), phone: Joi.string().regex(/^\\d{3}-\\d{3}-\\d{4}$/).required(), //”123-456-7890″ address: Joi.object({ street: Joi.string().min(3).required(), city: Joi.string().min(3).required(), state: Joi.string().min(3).required(), zip: Joi.number().min(3).required(), }).required(), hobbies: Joi.array().items(Joi.string()).required(), }).options({ abortEarly: false });
Properties in the userSchema are subject to the following constraints:
- Email: It must contain a valid email address.
- Password. The string must have a minimum of six characters.
- Age. A minimum of 18 is required.
- Employed. An optional boolean.
- Phone. This string must match the regex expression (/^\d{3}-\d{3}-\d{4}$/).
- Address. An object that represents a user’s address and its subproperties.
- Street. Strings must have a minimum length of three characters.
- City. There must be a minimum of three characters in the string.
- State. Three characters are required in the string.
- Zip. Three is a minimum required value.
- Hobbies. There is a string array required.
Besides setting the constraints, userSchema turns abortEarly off. When Joi encounters an error, it prints the error to the console and stops the execution of the program. When this option is set to false, Joi will examine the entire schema and print any errors that it encounters.
Validating Data With Joi
Put the userSchema code in validation.js.
So, for example:
// validation.js const Joi = require("joi"); const userSchema = Joi.object({ /* Your schema definition here */ }).options({ abortEarly: false }); module.exports = userSchema;
In the middleware, you will need to include the following code below the userSchema code as a method of intercepting request payloads and checking them against a schema.
// Middleware const validationMiddleware = (schema) => { return (req, res, next) => { const { error } = schema.validate(req.body); if (error) { // Handle validation error console.log(error.message); res.status(400).json({ errors: error.details }); } else { // Data is valid, proceed to the next middleware next(); } }; };
Middleware validates a request body when it is made using the validate method of the schema. Whenever a validation error occurs, the middleware responds with a 400 Bad Request message containing the details of the validation error.
When the validation has passed without errors, next() is called by the middleware.
Last but not least, export the validation middleware and the user schema.
module.exports = { userSchema, validationMiddleware, };
Testing Validation Constraints
Your router.js file should include validationMiddleware and userSchema. The middleware is set up as follows:
const { validationMiddleware, userSchema } = require("./validation"); router.post("/signup", validationMiddleware(userSchema), demoHandler);
The following command should be run to launch your application:
node index.js
Next, send an HTTP POST request to localhost:3000/signup using the following test data. This can be achieved using cURL or another API client.
{ "email": "user@example", // Invalid email format "password": "pass", // Password length less than 6 characters "age": 15, // Age below 18 "employed": true, "hobbies": ["reading", "running"], "phone": "123-456-789", // Invalid phone number format "address": { "street": "123", "city": "Example City", "state": "Example State", "zip": 12345 } }
There are a lot of invalid fields in the payload, including email, password, age, and phone, so this request would fail and return an error object. In order to handle errors appropriately, you can use the provided error object.
Conclusion
Here’s the basics of using Joi for data validation. Joi has more in-depth documentation on advanced techniques. JavaScript’s Joi simplifies data validation, giving you a simple, intuitive way to improve your app’s reliability and integrity.